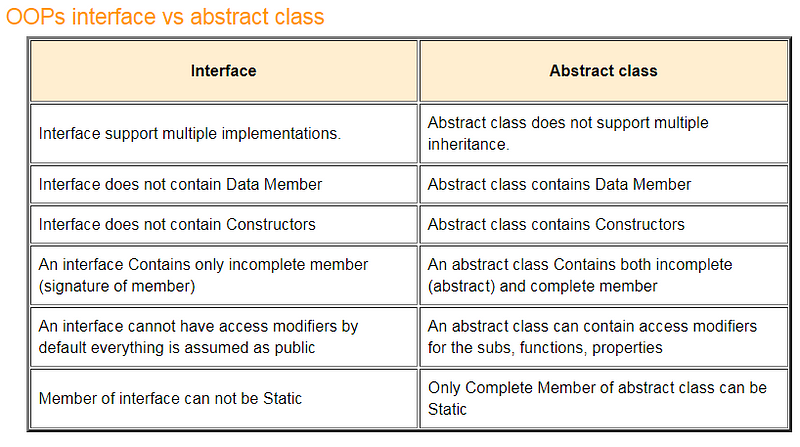
Create OG Image With PHP
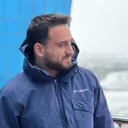
Adding text to an image using PHP can be crucial for various purposes, such as generating SEO images for Open Grapth images with post titles overlaid on a template background.
In this post, we will cover how to create an Open Graph image easily with using PHP.
Using the-og library
GitHub: https://github.com/simonhamp/the-og
The-og library is a powerful tool that allows developers to generate Open Graph images programmatically with PHP. It provides a simple interface for adding text, shapes and making it ideal for dynamically generating images for web content.
Installation
composer require simonhamp/the-og
Here's a basic example:
use SimonHamp\TheOg\Background;
use SimonHamp\TheOg\Image;
(new Image())
->accentColor('#cc0000')
->border()
->url('https://example.com/blog/some-blog-post-url')
->title('Some blog post title that is quite big and quite long')
->description(<<<'TEXT'
Some slightly smaller but potentially much longer subtext. It could be really long so we might need to trim it completely after many words.
Some slightly smaller but potentially much longer subtext. It could be really long so we might need to trim it completely after many words.
TEXT)
->background(Background::JustWaves, 0.2)
->save(__DIR__.'/test.png');
Writing Your Own Image Generator
It is not more than adding some text to an image. So you can use GDImage or Imagick to generate this type of image.
$title = $post->title ?? '';
$description = $post->meta_description ?? '';
$url = BASE_URL . "/{$post->slug}";
// Set the dimensions for the image
$width = 1280;
$height = 640;
// Create a new image with the specified dimensions
$image = \imagecreatetruecolor($width, $height);
// Load the background image
$imgPath = __DIR__ . "/../../public/images/og-bg.png";
$background = imagecreatefrompng($imgPath);
// Resize the background image to fit the canvas
imagecopyresampled($image, $background, 0, 0, 0, 0, $width, $height, imagesx($background), imagesy($background));
// Set text color to black
$textColor = imagecolorallocate($image, 0, 0, 0);
// Load font file
$font = __DIR__ . "/../../public/fonts/Inter/Inter-VariableFont_slnt,wght.ttf";
$boldFont = __DIR__ . "/../../public/fonts/Inter/static/Inter-Bold.ttf";
$semiboldFont = __DIR__ . "/../../public/fonts/Inter/static/Inter-SemiBold.ttf";
$titleX = 20;
$titleY = 175;
$descriptionX = 20;
$descriptionY = 250;
$urlX = 20;
$urlY = 100;
// Add text to the image
imagettftext($image, 16, 0, $urlX, $urlY, $textColor, $semiboldFont, $url);
imagettftext($image, 32, 0, $titleX, $titleY, $textColor, $boldFont, $title);
imagettftext($image, 20, 0, $descriptionX, $descriptionY, $textColor, $font, $description);
// Set content type header
header("Content-type: image/jpeg");
// cache the image for 30 days
header('Cache-Control: max-age=2592000');
header('Expires: ' . gmdate('D, d M Y H:i:s', time() + 2592000) . ' GMT');
// Output the image
imagejpeg($image);
// Free up memory
imagedestroy($image);
imagedestroy($background);
Do not forget to download the fonts and set your paths correctly.