
How to enable Firebase Crashlytics in Flutter
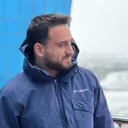
My notes about Firebase Crashlytics in the Flutter application.
Firebase Crashlytics is a crash reporting and analysis service provided by Google as part of the Firebase suite of app development tools.
It helps developers track, prioritize, and fix stability issues in mobile applications. When an app crashes, Firebase Crashlytics captures and logs information about the crash, providing developers with detailed reports to understand the root causes of the issues.
Firebase Crashlytics PROS
Firebase Crashlytics offers several advantages that streamline the process of identifying and resolving crashes in mobile applications.
Here’s an overview of key benefits:
- Efficient Debugging: Firebase Crashlytics accelerates debugging by providing clear and concise crash reports. The platform’s dashboard offers visual analytics, simplifying the identification of the root cause of crashes in interconnected application components.
- Insightful Custom Keys and Logs: Managing crashes at the user’s end can be challenging. Firebase Crashlytics addresses this by offering custom keys and logs that capture a snapshot of the moment when an issue occurs. This detailed information, including specific variable data, facilitates easier tracing and resolution of the problem.
- Breadcrumbs for Faster Reproduction: The Breadcrumbs feature automatically collects information about events leading up to a crash. This includes user interactions and, when combined with custom keys and logs, significantly eases the reproduction and understanding of the crash.
- Real-time Crash Report Notifications: Firebase Crashlytics provides real-time notifications for crash reports. Notifications can be customized based on the crash’s time and priority, ensuring efficient management.
In summary, Firebase Crashlytics enhances the development and maintenance process by offering features that simplify crash identification, debugging, and resolution.
The platform’s real-time capabilities and integration with visualization tools contribute to a more efficient and proactive approach to managing app stability.
How to enable your Flutter Project?
At to root of your project run;
flutter pub add firebase_crashlytics
Then run;
flutterfire configure
If you didn’t install FlutterFire CLI please check it out here.
After that run your application.
In the second step, we need to configure the crash handlers. Open your project main and override the FlutterError.onError with FirebaseCrashlytics.instance.recordFlutterFatalError.
Also for catching asynchronous errors use PlatformDispatcher.instance.onError.
Future<void> main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
FlutterError.onError = (errorDetails) {
FirebaseCrashlytics.instance.recordFlutterFatalError(errorDetails);
};
PlatformDispatcher.instance.onError = (error, stack) {
FirebaseCrashlytics.instance.recordError(error, stack, fatal: true);
return true;
};
runApp(MyApp());
}
To finish setting up Crashlytics and see initial data add this code anywhere in your application.
TextButton(
onPressed: () => throw Exception(),
child: const Text("Throw Test Exception"),
),
Then run this code block after the build. That is all. Then you will be able to see logs in your project.