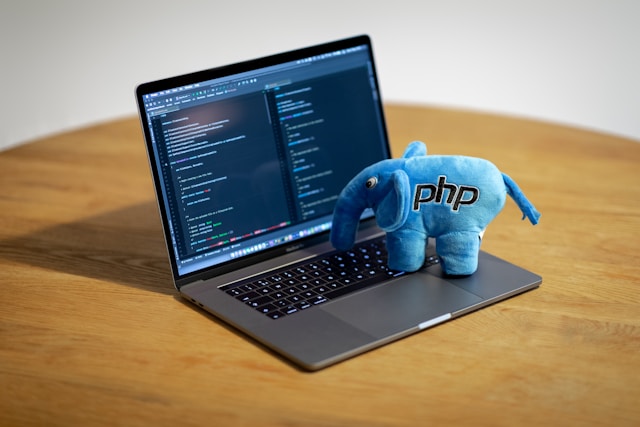
Laravel with React: A Step-by-Step Guide
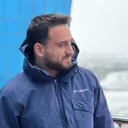
In this guide, we will create a Laravel application with React, and serve it under the /app
route.
Follow these steps to get started:
First, let’s create a new Laravel project using Composer:
composer create-project laravel/laravel laravel-react-example
2. Serve the Laravel Application
Navigate to the project directory and serve the application:
cd laravel-react-example
php artisan serve
You can now visit http://127.0.0.1:8000 to see the default Laravel page.
3. Install React and Required Packages
Open a new terminal tab and run the following commands to install React and other necessary packages:
pnpm i react
pnpm i react-dom
pnpm i react-router-dom
pnpm i @vitejs/plugin-react
Edit the Vite configuration file (vite.config.js
):
import { defineConfig } from 'vite';
import laravel from 'laravel-vite-plugin';
import react from '@vitejs/plugin-react';
export default defineConfig({
build: {
minify: process.env.APP_ENV === 'production' ? 'esbuild' : false,
cssMinify: process.env.APP_ENV === 'production',
},
plugins: [
laravel({
input: ['resources/react/App.jsx'],
refresh: true,
}),
react(
{
jsxRuntime: 'classic',
}
),
],
});
5. Create the Initial React Example
First, create a react
folder under the resources
directory. Then, create the App.jsx
file in the react
folder:
import React from 'react';
import ReactDOM from 'react-dom/client';
const MyApp = () => {
return (
<>Hello from MyApp</>
);
}
ReactDOM.createRoot(document.getElementById('app')).render(
<MyApp />
);
6. Set Up the Initial HTML Page
Create a new file named react.blade.php
under the resources/views
path:
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>{{ config("app.name") }}</title>
<link rel="shortcut icon" href="{{ asset('favicon.ico') }}" />
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Inter:wght@100..900&display=swap" rel="stylesheet">
@viteReactRefresh
@vite('resources/react/App.jsx')
</head>
<body>
<div id="app"></div>
</body>
</html>
7. Set Up Routing in Laravel
To use React Router, we need to render React for specific paths. Open the web.php
file under routes
and add the following:
Route::get('/app{any}', function () {
return view('react'); // this is pointing resources/views/react.blade.php
})->where('any', '.*');
8. Run the Development Servers
Run these commands in separate terminal tabs:
php artisan serve
pnpm dev
9. View Your React Application
Open http://127.0.0.1:8000/app/
in your browser. You should see the text "Hello from MyApp".
Following these steps, you’ve successfully set up a Laravel application with a React front-end served under the /app
route.