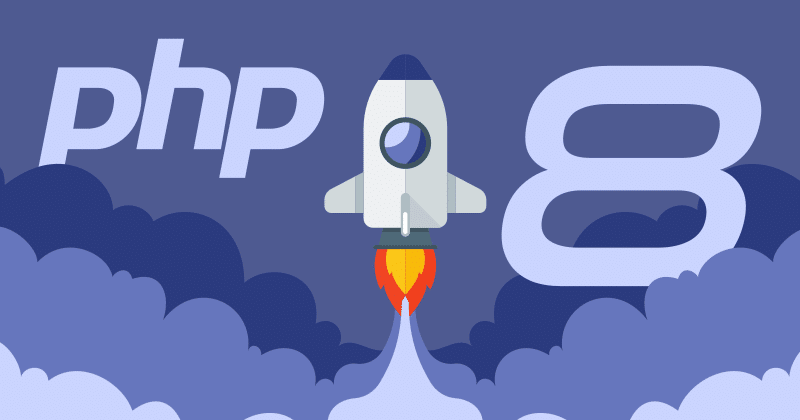
Best Practices for Secure PHP Code
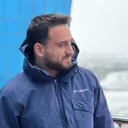
In today's digital world, security is more important than ever. PHP, being a widely used scripting language for web development, is often targeted by malicious actors. However, with the right strategies and practices, developers can protect their applications from common vulnerabilities. In this article, we will explore the best practices for writing secure PHP code.
Understanding PHP Security
PHP is not inherently insecure, but improper coding practices can lead to vulnerabilities. Understanding PHP security is the first step in securing your applications. There are several common vulnerabilities, including:
- SQL Injection
- Cross-Site Scripting (XSS)
- Cross-Site Request Forgery (CSRF)
- File Inclusion Vulnerabilities
- Session Hijacking
1. Use Prepared Statements for Database Queries
One of the most critical aspects of PHP security is preventing SQL Injection attacks. The best way to achieve this is by using prepared statements. Prepared statements ensure that user input is not executed as part of the SQL query.
$stmt = $pdo->prepare('SELECT * FROM users WHERE email = :email');
$stmt->execute(['email' => $email]);
2. Validate and Sanitize User Input
Always validate and sanitize all user inputs. Validation ensures the input meets the necessary criteria, while sanitization removes any potentially harmful characters.
$email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL);
For other inputs, use functions like htmlspecialchars()
to prevent XSS attacks.
3. Use CSRF Tokens
Cross-Site Request Forgery can be mitigated by using CSRF tokens. A CSRF token is a unique, secret value that is added to forms and verified on form submission, ensuring that the request is legitimate.
<input type="hidden" name="csrf_token" value="">
4. Keep PHP and Libraries Updated
Always keep your PHP version and its libraries up to date. Security patches are released regularly, fixing vulnerabilities that could be exploited if left outdated.
Secure Configuration of Your Server
In addition to secure PHP code, it’s vital to configure your server securely.
5. Disable Unused PHP Functions
Make sure to disable any PHP functions that are not needed for your application. This helps to prevent potential attacks. You can disable functions by adding them to the disable_functions
directive in your php.ini
file.
6. Set Appropriate File Permissions
Ensure that your files and directories have the correct permissions set. Typically, PHP files should be set to 644
and directories to 755
. Avoid setting permissions to 777
as it allows anyone to write to your files.
7. Use HTTPS
Always serve your application over HTTPS. This encryption protects data in transit, preventing sensitive information from being intercepted by attackers.
Implement Authentication and Authorization
Protecting your application’s endpoints is crucial.
8. Strong Password Hashing
Never store plain-text passwords. Use PHP’s built-in functions like password_hash()
and password_verify()
to hash and verify passwords.
$hashed_password = password_hash($password, PASSWORD_DEFAULT);
9. Implement User Session Management
Use secure session management practices. Regenerate session IDs upon login or privilege changes to prevent session fixation attacks.
session_regenerate_id(true);
Regular Security Audits
Regularly audit your code for vulnerabilities. Use tools like PHP CodeSniffer
or third-party services to scan for potential issues. Continuous security monitoring will help you catch vulnerabilities early.
Conclusion
Securing PHP applications requires diligence and best practices. By following these guidelines—using prepared statements, validating inputs, keeping your server secure, and implementing strong authentication measures—you can greatly reduce the risk of vulnerabilities in your applications. Remember, security is not a one-time task but an ongoing process.