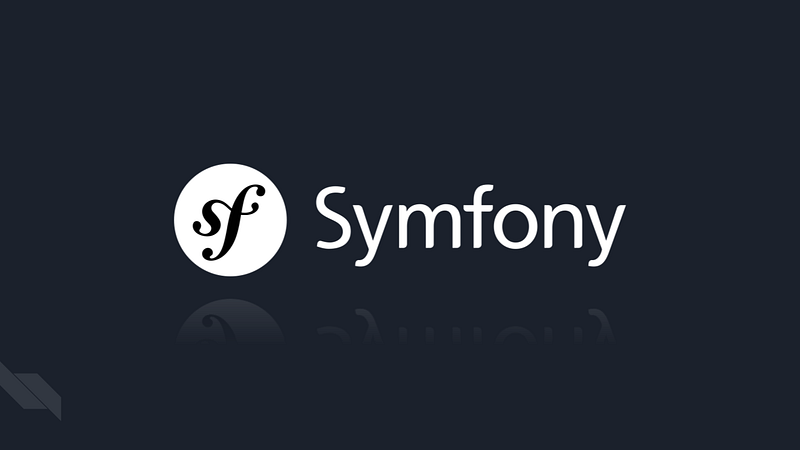
Laravel Collection Methods
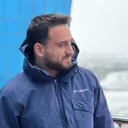
Laravel collections are a powerful feature of the Laravel framework that simplifies data manipulation and transformation.
They offer a fluent, convenient wrapper for working with arrays and are particularly useful when dealing with Eloquent models and other data sources.
In this guide, we'll explore key collection methods, demonstrate their use, and answer common questions related to Laravel collections.
Overview of Laravel Collection Methods
Laravel collections provide a wide array of methods that streamline data operations. These methods are available through the Illuminate\Support\Collection
class and can be applied to arrays or Eloquent collections. Some of the most commonly used methods include filter, map, reduce, sortBy, and groupBy.
Key Collection Methods
1. merge Method
The merge method allows you to combine two collections into one. By default, it merges the collections sequentially. However, you can merge by key to avoid overwriting values with matching keys.
$collection1 = collect(['name' => 'John', 'age' => 25]);
$collection2 = collect(['age' => 30, 'city' => 'New York']);
$merged = $collection1->merge($collection2); // Merges by key
2. sortBy Method
The sortBy method sorts the collection based on a given callback or key. This is particularly useful for organizing data in ascending order.
$collection = collect([['name' => 'Alice', 'age' => 22], ['name' => 'Bob', 'age' => 30]]);
$sorted = $collection->sortBy('age'); // Sorts by 'age'
3. random Method
The random method selects a random item from the collection. You can specify the number of items to retrieve.
$collection = collect([1, 2, 3, 4, 5]);
$randomItem = $collection->random(); // Retrieves a single random item
$randomItems = $collection->random(2); // Retrieves two random items
4. groupBy Method
The groupBy method groups the collection's items by a given key or callback. This is useful for categorizing items.
$collection = collect([
['name' => 'John', 'department' => 'HR'],
['name' => 'Jane', 'department' => 'Finance'],
['name' => 'Joe', 'department' => 'HR'],
]);
$grouped = $collection->groupBy('department'); // Groups by 'department'
Advanced Usage: Laravel Resource and Arrays
Laravel resources can accept multiple arrays or collections, enabling flexible data formatting for API responses.
$resource = new SomeResource($collection1, $collection2);
This feature allows combining and formatting data from different sources effectively.
Sorting with PHP's sortBy
When working with PHP collections, sorting can be achieved using Laravel's sortBy
method or native PHP functions like usort
.
$collection = collect([5, 2, 8, 1]);
$sorted = $collection->sort(); // Uses Laravel's sort
For custom sorting, use usort with a comparison function:
$array = [5, 2, 8, 1];
usort($array, function($a, $b) {
return $a - $b;
});
Conclusion
Laravel's collection methods offer powerful tools for manipulating and organizing data.
From merging collections by key to sorting and grouping data, these methods make it easier to handle complex data structures.
Whether you're working with Eloquent models or simple arrays, understanding these methods will enhance your ability to manage and present data efficiently.
Feel free to explore the official Laravel documentation for a comprehensive list of all collection methods and their usage. Happy coding!