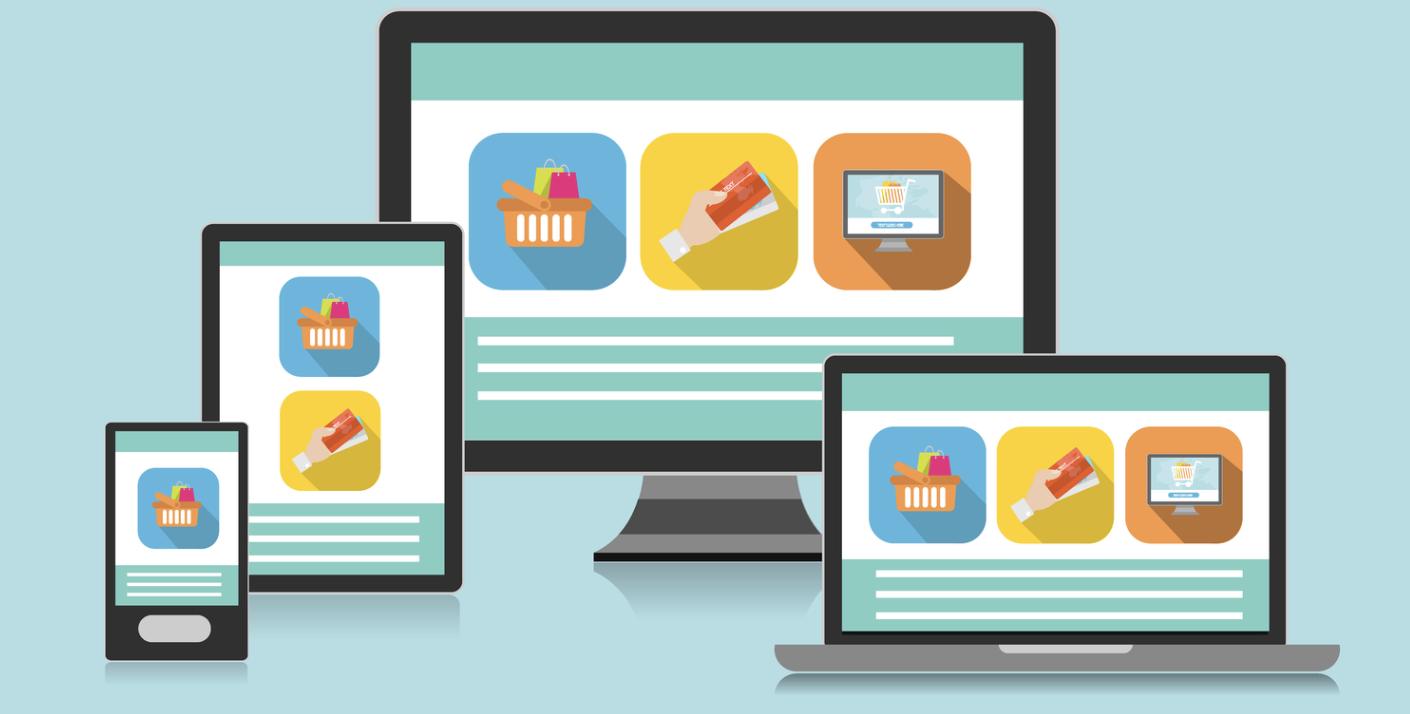
Laravel Collections Explained
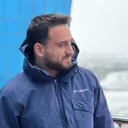
Elevate your PHP coding game with Laravel Collections, making array handling simpler and more powerful. This article unveils numerous quick tips to enhance your codebase immediately.
What is laravel collection?
Laravel Collections revolutionize array manipulation in PHP. They provide a fluent, chainable interface that wraps native array functions, adding new capabilities while ensuring immutability. Collections do not alter the original array but return a new one with modifications.
Ultimately, your code will become more readable and maintainable.
Initializing a Collection
To create a collection from an array, simply use the collect()
helper:
$collection = collect(); // Empty collection.
$collection = collect(["A", "B", "C"]); // Collection from an array.
// Alternativelly you can use in this way
use \Illuminate\Support\Collection;
$collection = Collection::make(["A", "B", "C"]);
Converting a Collection to an Array
Transform a collection back into an array with the toArray()
method:
$collection = collect([1, 2, 3]);
$array = $collection->toArray();
Iterating Over Collections
Though collections are designed to enhance array handling, they can still be used in foreach
loops:
foreach ($collection as $item) {
// Process each item
}
You can also retrieve keys:
foreach ($collection as $key => $value) {
// Process key-value pairs
}
Collections implement the IteratorAggregate
interface, making them iterable.
Replacing foreach
with each
Use the each
method to replace traditional foreach
loops:
$collection = collect(['Apple', 'Banana', 'Cherry']);
$collection->each(function ($value, $key) {
// Process each item
});
Merging Collections
Combining collections is straightforward with the merge()
method:
$fruits = collect(['Apple', 'Banana']);
$berries = collect(['Strawberry', 'Blueberry']);
$allFruits = $fruits->merge($berries);
Filtering Collections
Use the filter()
method to clean up collections:
$collection = collect([1, 2, 3, 4, 5]);
$filtered = $collection->filter(function ($value) { return $value > 2; });
Mathematical Operations
Collections provide methods for common mathematical operations:
$numbers = collect([1, 2, 3, 4, 5]);
$sum = $numbers->sum(); // 15
$average = $numbers->average(); // 3
$min = $numbers->min(); // 1
$max = $numbers->max(); // 5
Higher Order Messages
Simplify your code with higher-order messages:
User::where('active', true)->get()->each->notify(new Notification);
Key Management with only
and except
Retain specific keys using only()
:
$original = ['name' => 'John', 'age' => 30, 'city' => 'New York'];
$filtered = collect($original)->only(['name', 'city']);
Exclude specific keys with except()
:
$filtered = collect($original)->except(['age']);
Random Element Selection in Laravel Collection
You can select a random element using random()
:
$collection = collect(['Red', 'Green', 'Blue']);
$randomColor = $collection->random();
Mapping Without Temporary Variables
Transform collections with map()
:
$collection = collect([1, 2, 3]);
$transformed = $collection->map(function ($value) {
return $value * 2;
});
How to check if the Laravel collection is empty or not?
Check if a collection is empty or not:
if ($collection->isEmpty()) {
// Collection is empty
}
if ($collection->isNotEmpty()) {
// Collection is not empty
}
Conditional Transformations
Apply conditional logic with when()
and unless()
:
$collection = collect([1, 2, 3]);
$filtered = $collection->when(true, function ($collection) {
return $collection->filter(function ($value) {
return $value > 1;
});
});
Incorporating these tips will not only make your code more efficient but also enhance readability and maintainability. Explore Laravel Collections to harness their full potential and transform your PHP development experience.