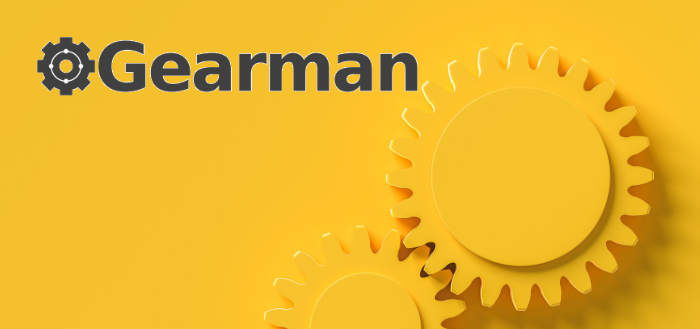
Published in PHP
How To Secure PHP Sessions?
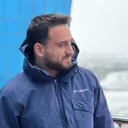
By Atakan Demircioğlu
Fullstack Developer
My notes about how to secure PHP Sessions, what is session hijacking, and so on.
Session Hijacking
- The attacker steals the active session of the user.
Session Fixation
- Imagine the attacker steals your session ID while in the Guest. Then you log in to your account. If the application doesn’t generate the session id again, you give direct auth to the attacker.
Defend Session attacks in PHP
- IP match control
- User-agent control
- Limit the access, if the last access is more than x hours, destroy the session.
- Configure cookie options (secure, httponly, samesite)
- To prevent session fixation, use the session_regenerate_id() function after login.
- Apply guest middleware
Setting HTTP Only Cookies In PHP
- Setting HTTP only true provides to prevent access on the client side that cookies if the browser supports it.
ini_set( 'session.cookie_httponly', 1 );
OR (PHP 8 +)
setcookie( $name, $value, httponly:true )
OR
session_start(['cookie_httponly' => true]);
Setting Secure Cookies In PHP
- The browser will not send a cookie with the secure attribute set over an unencrypted HTTP request.
session.cookie_secure = True
OR set it true with the set session_set_cookie_params function
session_set_cookie_params([
'lifetime' => 'YOUR_LIFE_TIME_VALUE',
'path' => 'YOUR_PATH',
'domain' => 'YOUR_DOMAIN',
'secure' => true, // set it true for secure
'httponly' => true, // set it true for secure
'samesite' => 'YOUR_SAME_SITE_VALUE'
]);
Same Site Cookie In PHP
- HTTP response header allows you to declare if your cookie should be restricted to a first-party or same-site context.
Edit with session_set_cookie_params function. (Lax, Strict, etc.)
For a better understanding of the types of the same site attributes, please visit this link.
ini_set(‘session.cookie_samesite’, ‘HERE_WRITE_VALUE’);
OR (you can set it with this function setting up the options)