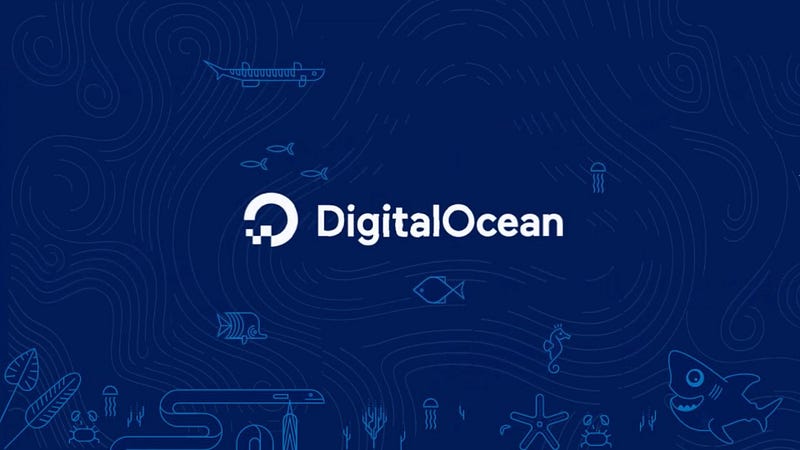
Generate Sitemap In Symfony 6
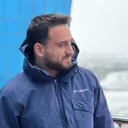
My notes about generating a sitemap in a Symfony 6 application.
There are two main ways to implement a sitemap feature for your application.
- Using a bundle
- Creating your custom sitemap
If you are using the first way, there are some great bundles for creating a sitemap in Symfony.
Note: I will update this list if I find a better bundle.
If you are using the second option, let's code a sitemap controller for our application.
First step: Create a controller named SitemapController.php under src/Controller
<?php
namespace App\Controller;
use App\Repository\PostRepository;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Routing\Annotation\Route;
use Symfony\Component\Routing\Generator\UrlGeneratorInterface;
class SitemapController extends AbstractController
{
public function __construct(PostRepository $blogPostRepository)
{
$this->blogPostRepository = $blogPostRepository;
}
#[Route('/sitemap.xml', name: 'sitemap')]
public function index()
{
// find published blog posts from db
$posts = $this->blogPostRepository->findBy(['published' => 1]);
$urls = [];
foreach ($posts as $post) {
$urls[] = [
'loc' => $this->generateUrl(
'post',
['slug' => $post->getSlug()],
UrlGeneratorInterface::ABSOLUTE_URL
),
'lastmod' => $post->getUpdatedAt()->format('Y-m-d'),
'changefreq' => 'weekly',
'priority' => '0.5',
];
}
$response = new Response(
$this->renderView('./sitemap/sitemap.html.twig', ['urls' => $urls]),
200
);
$response->headers->set('Content-Type', 'text/xml');
return $response;
}
}
In the constructor, let's pass the repository that you need to query, and in the index method just convert them to sitemap format.
You are free to change some settings like changefreq or etc.
In the last step create a twig template under the templates directory.
<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9" xmlns:xhtml="http://www.w3.org/1999/xhtml"
xmlns:image="http://www.google.com/schemas/sitemap-image/1.1" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.sitemaps.org/schemas/sitemap/0.9 http://www.sitemaps.org/schemas/sitemap/0.9/sitemap.xsd">
{% for url in urls %}
<url>{# check if hostname is not alreay in url#}
<loc>{{ url.loc }}</loc>
{% if url.lastmod is defined %}
<lastmod>{{url.lastmod}}</lastmod>
{% endif %}
{% if url.changefreq is defined %}
<changefreq>{{url.changefreq}}</changefreq>
{% endif %}
{% if url.priority is defined %}
<priority>{{url.priority}}</priority>
{% endif %}
</url>
{% endfor %}
</urlset>
That’s all, you can now check your sitemap your-website/sitemap.xml .