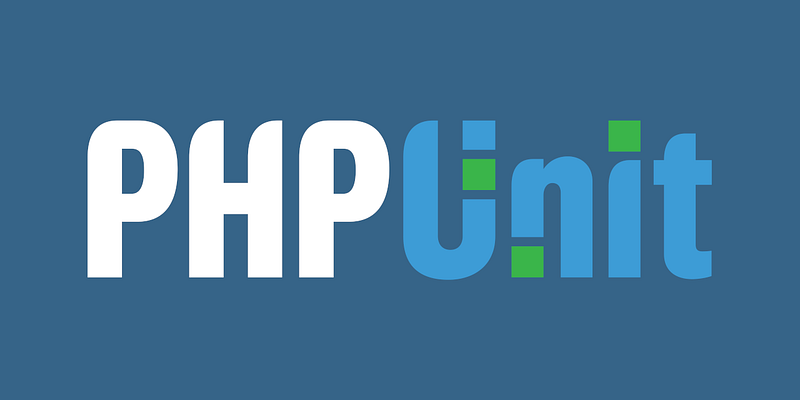
Understanding PHP in_array: A Comprehensive Guide
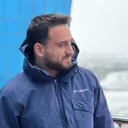
Introduction
PHP, a widely-used open-source scripting language, excels in web development and can be embedded into HTML. One of the many powerful functions in PHP is in_array
. This function is used to check if a value exists in an array. Understanding how to use in_array
efficiently can greatly enhance your ability to manage and manipulate data in your PHP applications.
What is in_array
?
The in_array
function searches an array for a specific value and returns true
if the value is found in the array, and false
otherwise. This function is particularly useful when you need to verify the presence of an element in an array before performing operations based on its existence.
Syntax
in_array(mixed $needle, array $haystack, bool $strict = false): bool
- $needle: The value to search for in the array.
- $haystack: The array to search in.
- $strict: (Optional) If set to
true
, thein_array
function will also check the types of the $needle and the elements in the $haystack.
Parameters
- $needle: This is the value you are searching for in the array.
- $haystack: This is the array in which you are searching for the needle.
- $strict: This optional parameter, if set to
true
, enforces a strict comparison of the $needle and the array elements, checking both the value and the type.
Basic Usage
Here's a simple example to illustrate the basic usage of in_array
:
$fruits = array("apple", "banana", "orange");
if (in_array("banana", $fruits)) {
echo "Banana is in the array!";
} else {
echo "Banana is not in the array.";
}
In this example, the in_array
function checks if the string "banana" exists in the $fruits
array. Since "banana" is in the array, the function returns true
, and the message "Banana is in the array!" is displayed.
Using in_array
with the Strict Parameter
The strict
parameter is useful when you need to ensure that the types match as well as the values. This can prevent unexpected results when working with mixed data types.
$numbers = array(1, 2, "3");
if (in_array(3, $numbers, true)) {
echo "The number 3 is in the array.";
} else {
echo "The number 3 is not in the array.";
}
In this case, in_array
will return false
because the strict parameter is set to true
, and while "3" (string) is in the array, the integer 3 is not.
Practical Examples
Checking User Roles
Imagine you have an array of user roles, and you want to check if a specific user has a certain role.
$user_roles = array("admin", "editor", "subscriber");
if (in_array("admin", $user_roles)) {
echo "User is an admin.";
} else {
echo "User is not an admin.";
}
Validating Form Input
You can use in_array
to validate if a selected option from a dropdown menu is valid.
$valid_options = array("red", "blue", "green");
$selected_option = $_POST['color'];
if (in_array($selected_option, $valid_options)) {
echo "Valid color selected.";
} else {
echo "Invalid color selected.";
}
Performance Considerations
While in_array
is quite efficient for small arrays, its performance can degrade with larger arrays due to its linear search algorithm. If you need to perform frequent existence checks on large datasets, consider using more efficient data structures like associative arrays or implementing binary search for sorted arrays.
Faster Alternatives to in_array
When working with larger datasets or requiring frequent checks for existence, there are faster alternatives to using in_array
. Here are some methods to enhance performance:
Using Associative Arrays
Associative arrays (or hash tables) provide constant time complexity (O(1)) for lookups, making them significantly faster than in_array
for large arrays.
$values = array_flip(array("apple", "banana", "orange"));
if (isset($values["banana"])) {
echo "Banana is in the array!";
} else {
echo "Banana is not in the array.";
}
In this example, array_flip
converts the values to keys in an associative array, and isset
checks for the existence of the key, which is much faster than in_array
.
Conclusion
The in_array
function is a versatile and essential tool in PHP for checking the existence of a value within an array. Whether you're validating form inputs, checking user roles, or simply searching for values, in_array
provides a straightforward and efficient solution. By understanding its syntax, parameters, practical applications, and faster alternatives, you can harness the full power of in_array
to enhance your PHP development.
Happy coding!