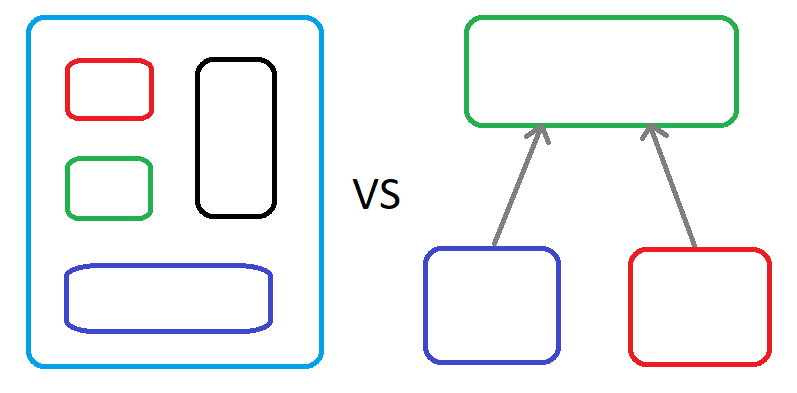
What are array_reduce and array_walk in PHP?
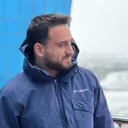
I liked to use array_reduce and array_walk in PHP. These are my notes about array_reduce and array_walk.
What is array_reduce?
If you are coming from the JS side, mostly you know the reduce() function. I think it is popular on the JS side.
When I look at PHP documents there is a short description of it;
Iteratively reduce the array to a single value using a callback function
Syntax:
array_reduce(array, callback, initial)
Parameters:
- array: It is the input array that will be reduced to a single value.
- callback: It is a callback function that determines how the array should be reduced.
- initial: It is an optional value that will be used at the beginning of the process, or as a final result in case the array is empty.
Example:
$a = [1, 2, 3, 4, 5, 6];
$num1 = array_reduce($a, function ($num1, $num2) {
return $num1 + $num2;
}, 0);
echo $num1;
// This will print out 21
// Alternative with foreach
$a = [1, 2, 3, 4, 5, 6];
$result = 0;
foreach ($a as $value) {
$result += $value;
}
var_dump($value); // this will print out 6
echo $result;
// This will print out 21
When I compare with foreach, I think in heavy calculations foreach can be faster. I agree with this.
But for similar cases like this example, doing this with foreach has some disadvantages. For example, you can print the last value from outside of the foreach loop. Also, I don’t like generally to mutate a variable.
What is array_walk?
Applies the user-defined callback function to each element of the array array.
- The array’s keys and values are parameters in the function
- The array_map() cannot operate with the array keys, while the array_walk() function can work with the key values pair.
- Returns true
- Advantages to use array_walk are clarity, type-hinting, and scoping.
Syntax:
array_walk(array, callback, parameter...)
Parameters:
- array: The input array.
- callback: Name of the function
- parameter: Specifies a parameter to the user-defined function. You can assign multiple parameters.
Example:
$a = ["a" => "red", "b" => "green", "c" => "blue"];
array_walk($a, function ($value, $key) {
echo sprintf("The key %s has the value %s", $value, $key) . PHP_EOL;
});
// The key red has the value a
// The key green has the value b
// The key blue has the value c
Example 2:
$arr = array(1, 2, 3, 4);
foreach ($arr as &$value) {
$value = $value * 2;
}
var_dump($arr); // array(2, 4, 6, 8)
unset($value); // break the reference with the last element
$arr = array(1, 2, 3, 4);
array_walk($arr, function (&$value) {
$value = $value * 2;
});
var_dump($arr); // array(2, 4, 6, 8)
// no reference with last element is present
Summary
There is no one way to do whole array iterations and operations. Clarity is the key point. If your code is getting more complex with using these functions, don’t use it. If it is getting more clear, just use it.
References
- https://gist.github.com/Potherca/640471e0e9918b803feb5d1b0ff788ef
- https://www.php.net/manual/en/function.array-walk.php
- https://www.geeksforgeeks.org/what-are-array_map-array_reduce-and-array_walk-function-in-php/
- https://stackoverflow.com/questions/3432257/difference-between-array-map-array-walk-and-array-filter