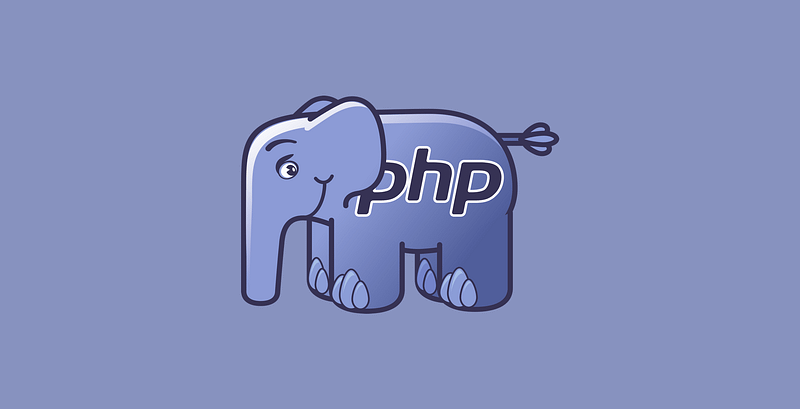
Understanding PHP’s declare(strict_types=1): A Comprehensive Guide
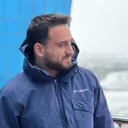
In the world of PHP programming, managing code quality and predictability is crucial. One powerful feature that PHP offers to improve type safety is the declare(strict_types=1) directive.
This guide will explore the significance of declare(strict_types=1), its impact on type hinting, and how it integrates with frameworks like Laravel.
What is declare(strict_types=1)?
The declare(strict_types=1) directive is a PHP feature that enforces strict type-checking in your scripts.
By default, PHP performs type coercion, where it attempts to convert types automatically when they don’t match.
For example, PHP will automatically do so if a function expects an integer but receives a string that can be converted to an integer.
Adding declare(strict_types=1) at the beginning of a PHP file changes this behavior.
It forces PHP only to accept the specified types and throws an TypeError if the types do not match exactly. This directive can help catch bugs early by ensuring that type mismatches are detected during development rather than at runtime.
How to Use declare(strict_types=1)
To activate strict typing, you need to place the declare(strict_types=1)
directive at the top of your PHP file before any other code:
<?php
declare(strict_types=1);
function addNumbers(int $a, int $b): int {
return $a + $b;
}
echo addNumbers(5, '10'); // This will throw a TypeError
?>
In the example above, the addNumbers function is strictly typed to accept integers only.
If you try to pass a string that can be converted to an integer, PHP will raise a TypeError due to the strict type of enforcement.
Why Use Strict Types in PHP?
Better Type Safety: Strict typing reduces unexpected behavior by ensuring that functions and methods receive and return values of the exact types specified. This minimizes type-related bugs and makes the code more predictable.
Improved Code Quality: When using strict types, you’re forced to be explicit about the types of variables, function parameters, and return values. This leads to better-designed code that adheres to clear contracts.
Easier Debugging: By catching type errors at the point of occurrence, you can quickly identify and fix issues, leading to a smoother development experience and more robust applications.
Integrating Strict Types with Type Hinting
Type hinting is another feature in PHP that works hand-in-hand with declare(strict_types=1). Type hints specify the expected data types for function arguments and return values. When strict typing is enabled, type hints become even more powerful:
<?php
declare(strict_types=1);
function concatenateStrings(string $a, string $b): string {
return $a . $b;
}
echo concatenateStrings('Hello, ', 'world!'); // Works fine
echo concatenateStrings('Hello, ', 123); // Throws a TypeError
?>
Using Strict Types in Laravel
Laravel, a popular PHP framework, does not require declare(strict_types=1) by default, but using it can still be beneficial. When integrating strict typing into Laravel applications, you can ensure that your controllers, models, and other components adhere to strict type checks, improving overall reliability.
For instance, in a Laravel controller method:
<?php
declare(strict_types=1);
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function store(Request $request): \Illuminate\Http\Response
{
// Method implementation
}
}
?>
Applying strict types in Laravel ensures that any type mismatch in request handling or other operations is immediately flagged, helping to maintain high code quality.
Conclusion
The declare(strict_types=1) directive is a valuable tool for PHP developers looking to enhance their code’s reliability and maintainability. By enforcing strict type checks, you ensure that your code adheres to defined contracts, reducing bugs and improving overall application quality. Whether you’re working on a simple PHP script or a complex Laravel application, integrating strict types and type hinting can lead to more predictable and robust code.