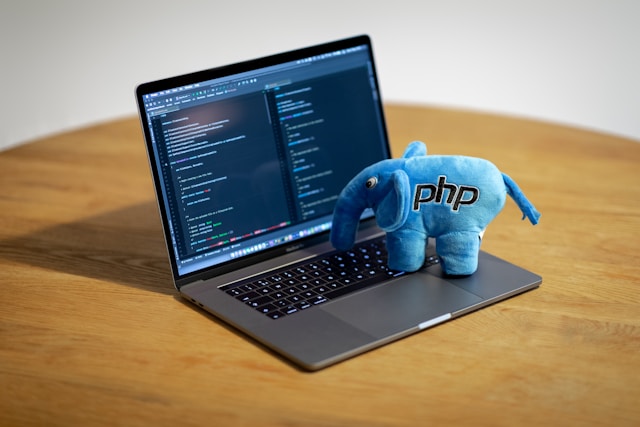
Template Method in PHP
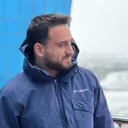
Whether you're a budding PHP developer or an experienced programmer, understanding the Template Method can be a game-changer for your coding practices.
So, let's dive straight into it!
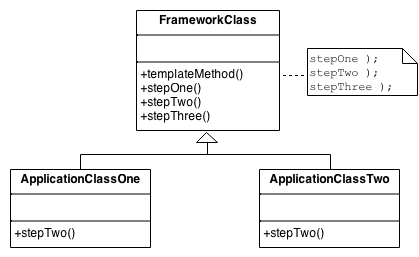
What is the Template Method?
The Template Method is a behavioral design pattern that outlines an algorithm's skeleton in a method called the template method. This method lets subclasses redefine certain steps of an algorithm without changing the algorithm's structure.
Template Method Example in PHP
Let's imagine you're organizing a grand dinner at home.
The two main courses are meat and vegan dishes. Although the cooking process for both is the same, their ingredients differ. This situation presents an excellent illustration of the Template Method.
Think of your cooking process as the algorithm. First, you get the ingredients (some are specific to meat, others to a vegan dish), prepare the ingredients, cook them, and finally serve the dish. This step sequence remains the same, but some steps are carried out differently depending on the dish.
// Our abstract class for the cooking process
abstract class Dinner {
// The template method
final function makeRecipe() {
$this->getIngredients();
$this->prepareIngredients();
$this->cook();
$this->serve();
}
abstract protected function getIngredients();
abstract protected function prepareIngredients();
abstract protected function cook();
abstract protected function serve();
}
// Concrete class for the meat dish
class MeatDish extends Dinner {
protected function getIngredients() {
echo "Getting ingredients for meat dish...\n";
}
protected function prepareIngredients() {
echo "Preparing ingredients for meat dish...\n";
}
protected function cook() {
echo "Cooking meat dish...\n";
}
protected function serve() {
echo "Serving meat dish...\n";
}
}
// Concrete class for the vegan dish
class VeganDish extends Dinner {
protected function getIngredients() {
echo "Getting ingredients for vegan dish...\n";
}
protected function prepareIngredients() {
echo "Preparing ingredients for vegan dish...\n";
}
protected function cook() {
echo "Cooking vegan dish...\n";
}
protected function serve() {
echo "Serving vegan dish...\n";
}
}
// Example usage
$meatDish = new MeatDish();
$meatDish->makeRecipe();
$veganDish = new VeganDish();
$veganDish->makeRecipe();
Also if you are interested in Design Patterns, please check out these articles;