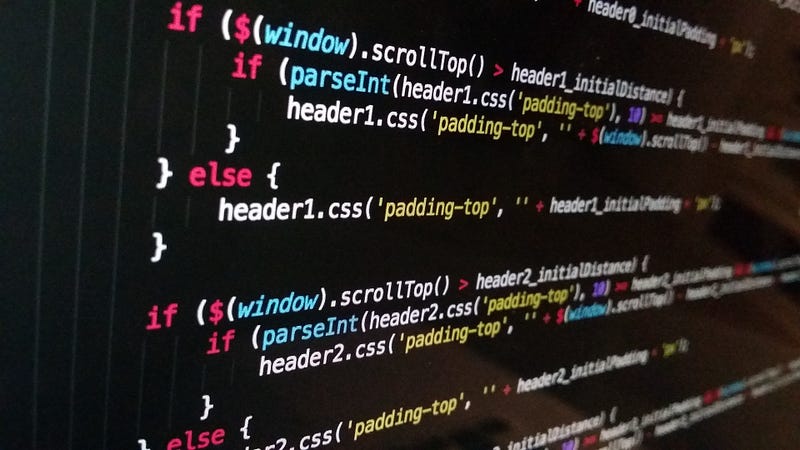
Design Patterns # Creational
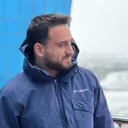
Here are my notes about creational design patterns with PHP examples.
1- Singleton Pattern: Involves a single class that is responsible to create an object while making sure that only a single object gets created.
- Singleton is an anti-pattern because it is hard testability. For better testability and maintainability use dependency injection.
- Examples: DB Class, Logger and etc.
2- Builder Pattern: The main purpose of the Builder design pattern is to separate the construction of a complex object from its representation.
- Allows producing different types and representations of an object using the same construction code.
- The director controls the building and specifies the parts and variations of an object
- This prevents the constructors of a class from having a long list of arguments
- Prevents having a lot of different constructs (PHP lacks support for declaring multiple constructors of different numbers of parameters for a class)
- There is a great article with Java examples here.
3- Simple Factory Pattern: A basic factory class that has a method and this method returns different types of objects based on the given input.
- Usually represented by a single method in a single class.
- It differs from the static factory because it is not static.
4- Abstract Factory Pattern: This lets you produce families of related objects without specifying their concrete classes.
- A super-factory that creates other factories.
- Tight coupling between concrete products and client code.
- The products that you’re getting from a factory are compatible with others.
5- Static Factory Pattern: Used to create a series of related or dependent objects.
- Static factory pattern uses just one static method to create all types of objects it can create. It is usually named factory or build
6- Factory Method Pattern: Known as Virtual Constructor.
This pattern provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.
- Achieves the Dependency Inversion principle
- Factory Method depends on abstractions, not concrete classes
7- Prototype Design Pattern: The main purpose of this pattern is to avoid the cost of creating new objects. With this pattern, we are trying to clone an object instead of creating a new one.
8- Pool Design Pattern: The main purpose of this pattern is storing initialized objects in a pool and when they are ready just use these ones. This avoids allocating and destroying costs.
- DB connections, socket connections, and threads are some examples.
You can check a worker example with this gist.
Also, you can check this example.
References:
Recommended Articles: