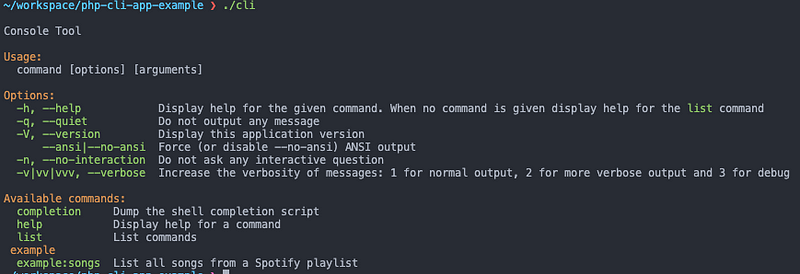
Test PHP codes with PHPUnit
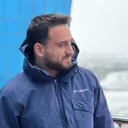
How to test PHP codes with PHPUnit. Testing with php.
Installing PHPUnit via composer;
composer require — dev phpunit/phpunit
Create a phpunit.xml file and define your settings and also your test unit.
Example of a phpunit.xml file
phpunit.xml
Line 5: This attribute configures whether colors are used in the output.
Line 10: Test suite execution should be stopped after the first test is finished with the status “failure”.
Line 14: This will run tests under ./tests/ directory.
You can find all settings here.
Adding Tests
As I mentioned, in our case you need to add your test files under the ./tests/ directory.
For testing, create a file named ValidatorTest.php and then create a class like this. You need to extend TestCase.
final class ValidatorTest extends TestCase {}
Setting Up Test
If you need to set up a class or a different thing you can use the setUp() method.
protected function setUp()
{
$this->validator = new Validator;
}
Adding an Example Test
public function testNumberIsEqual()
{
$myFirstNumber = 1;
$mySecondNumber = 1;
$this->assertTrue($myFirstNumber === $mySecondNumber);
}
In this example, I am checking if the numbers are equal. In this case, they are equal and this comparison will return true. And we are asserting true results. So, the test will pass.
In PHPUnit, there are a lot of different assertions.
Some examples are;
assertFalse, assertTrue, assertEquals, assertArrayHasKey ...
You can check from here.
Running Test Via Composer
We can add the “test” param in the scripts part inside composer.json.
"scripts": {
"test": "./vendor/bin/phpunit",
}
Then just need to run the “composer test” command to run tests.
Parameterized Test In PHPUnit
We can do this by using dataProvider.
/**
* @dataProvider provider
*/
public function testAdd($params, $rules)
{
$this->validator->validate($params, $rules);
$this->assertTrue($this->validator->passes());
}
public function provider()
{
return [
[['myInput' => 'required|string'], ['myInput' => 'my string']],
[['myInput' => 'required|numeric'], ['myInput' => '123']],
];
}
This is a basic example. We are sending multiple parameters to the provider and running tests with different parameters more than one time.
For checking some examples, you can check this repository.